In this article, I will discuss how to check the internet connectivity status globally in Flutter App.
I will use connectivity plus package to handle the connection status and will also use the Getx package to manage the states.
First, create a controller class name network_controller.dart
where we will implement the connectivity logic.
// network_controller.dart
import 'dart:async';
import 'package:connectivity_plus/connectivity_plus.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'dart:developer' as developer;
class NetworkController extends GetxController {
var isOnline = true.obs;
final Connectivity _connectivity = Connectivity();
late StreamSubscription<List<ConnectivityResult>> _connectivitySubscription;
@override
void onInit() {
super.onInit();
initConnectivity();
}
Future<bool> isInternetAvailable() async {
late List<ConnectivityResult> result;
try {
result = await _connectivity.checkConnectivity();
} on PlatformException catch (e) {
developer.log('Couldn\\'t check connectivity status', error: e);
return false;
}
return result.contains(ConnectivityResult.none) ? false : true;
}
Future<void> initConnectivity() async {
late List<ConnectivityResult> result;
try {
result = await _connectivity.checkConnectivity();
} on PlatformException catch (e) {
developer.log('Couldn\\'t check connectivity status', error: e);
return;
}
_connectivitySubscription = _connectivity.onConnectivityChanged.listen((result) {
_updateConnectionStatus(result);
});
}
Future<void> _updateConnectionStatus(List<ConnectivityResult> result) async {
if (result.contains(ConnectivityResult.none)) {
isOnline.value = false;
Get.rawSnackbar(
messageText: const Text(
'PLEASE CONNECT TO THE INTERNET',
style: TextStyle(
color: Colors.white,
fontSize: 14,
),
),
isDismissible: false,
duration: const Duration(days: 1),
backgroundColor: Colors.red[400]!,
icon: const Icon(Icons.wifi_off, color: Colors.white, size: 35),
margin: EdgeInsets.zero,
snackStyle: SnackStyle.grounded,
);
} else {
if (Get.isSnackbarOpen) {
Get.closeCurrentSnackbar();
isOnline.value = true;
}
}
}
@override
void onClose() {
_connectivitySubscription.cancel();
super.onClose();
}
}
Then we will use another class name dependency_injection.dart
class where we will initialize the NetworkController
class from our main.dart
file.
// dependency_injection.dart
import 'package:get/get.dart';
import 'network_controller.dart';
class DependencyInjection {
static void init() {
Get.put<NetworkController>(NetworkController(), permanent: true);
}
}
Now we will use it on main.dart
file after initializing our application.
//main.dart
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:get_storage/get_storage.dart';
import 'controllers/utils/dependency_injection.dart';
import 'routes/route.dart';
import 'views/splash/splash_screen.dart';
void main() async{
await GetStorage.init();
runApp(const MyApp());
DependencyInjection.init();
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return GetMaterialApp(
initialRoute: Routes.initialRoute,
debugShowCheckedModeBanner: false,
getPages: Routes.pages,
title: 'Network Connectivity App',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
darkTheme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
themeMode: ThemeMode.system,
home: SplashScreen(),
);
}
}
**[N.B]: Now we need to wrap the MaterialApp widget by the GetMaterialApp shown on the above code.**
Now, run the app and turn off the network(wifi/mobile data) you will see a red snack bar pop up and if you connect to the internet again the error message will immediately disappear.
See the screenshots below.
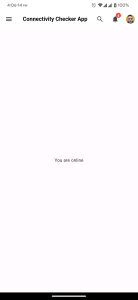
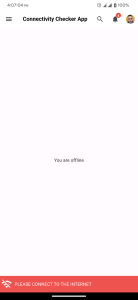